How Many Radio Buttons From the Following Code Can Be Selected at Any Given Time?
Summary: in this tutorial, you'll learn how to create a form with radio buttons and handle radio groups in PHP.
Introduction to radio buttons
To create a radio button, you use the <input>
element with the type radio
. For instance:
<input type="radio" name="contact" id="contact_email" value="e-mail" />
Lawmaking language: HTML, XML ( xml )
A radio button doesn't have a label. Therefore, y'all should always utilize a radio button with a <label>
chemical element similar this:
<input blazon="radio" name="contact" id="contact_email" value="e-mail"/> <label for="contact_email">Email</characterization>
Code language: HTML, XML ( xml )
To associate a radio button with a <characterization>
element, the value of the for
attribute of the characterization needs to exist the same equally the value of the id
of the radio button.
A radio button has two states: checked and unchecked.
When you link a label with a radio button, you tin can check the radio push by clicking the label or the radio button itself. Hence, the label increases the usability of the radio push because it expands the selection area.
Alternatively, yous can place the radio button within a <label>
chemical element similar this:
<label> <input blazon="radio" proper name="contact_email" value="email"> Electronic mail </label>
Lawmaking linguistic communication: HTML, XML ( xml )
In this instance, the radio links to the label without matching the for and id attributes.
To select a radio push button automatically when the page loads for the first time, you can use the checked
Boolean attribute:
<input type="radio" name="contact" id="contact_email" value="electronic mail" checked /> <characterization for="contact_email">Electronic mail</label>
Lawmaking language: HTML, XML ( xml )
Define a radio group
In practice, you ofttimes utilize the radio buttons in a group. A group of radio buttons is called a radio group. A radio group allows you to select simply one radio push at a time.
If you select any radio button in a group, the currently-selected radio button in the same grouping is automatically deselected.
To ascertain a radio grouping, you assign the same proper name to all the radio buttons in the same group.
The post-obit example defines a radio grouping that consists of ii radio buttons.
<input type="radio" proper name="contact" id="contact_email" value="email" /> <label for="contact_email">Email</label> <input blazon="radio" proper noun="contact" id="contact_phone" value="phone" /> <label for="contact_phone">Telephone</characterization>
Code linguistic communication: HTML, XML ( xml )
Handle radio buttons in PHP
When a class has a radio button with a name, you can get the checked radio button past accessing either $_POST
or $_GET
array, depending on the request method.
If a radio button is not checked, the $_POST
or $_GET
does not comprise the cardinal of the radio button. Therefore, you demand to use the isset()
role to bank check whether a radio button is checked or not:
isset($_POST['radio_name'])
Code language: PHP ( php )
Alternatively, you can use the filter_has_var()
part:
filter_has_var(INPUT_POST, 'radio_name')
Lawmaking language: JavaScript ( javascript )
The filer_has_var()
returns true
if it finds the radio button name in the INPUT_POST
.
If a radio button is checked, you lot get the value of the radio button from the $_POST
using the radio push name:
$_POST['radio_name']
Code language: PHP ( php )
PHP radio button example
Nosotros'll create a uncomplicated form with a radio group. If you do non select whatever option and submit the form, it'll bear witness an fault bulletin. Otherwise, information technology'll show the value of the selected radio button.
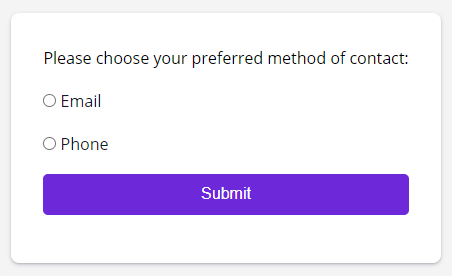
First, create the post-obit directory and file structure:
Lawmaking language: plaintext ( plaintext )
. ├── css | └── style.css ├── inc | ├── footer.php | ├── go.php | ├── header.php | └── post.php └── alphabetize.php
File | Directory | Clarification |
---|---|---|
index.php | . | Contain the primary logic that loads get.php or post.php depending on the HTTP request method |
header.php | inc | Contain the HTML header |
footer.php | inc | Comprise the HTML footer |
go.php | inc | Contain the code for showing a grade with radio buttons when the HTTP asking is GET. |
post.php | inc | Contain the code for handling POST request |
style.css | css | Contain the CSS code |
Second, add the following lawmaking to the header.php
file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <championship>PHP Radio Button</title> <link rel="stylesheet" href="css/mode.css"> </head> <trunk> <principal>
Code language: HTML, XML ( xml )
Third, add the following code to the footer.php
file:
</master> </torso> </html>
Code linguistic communication: HTML, XML ( xml )
Fourth, add together the following code to the alphabetize.php
file:
<?php crave __DIR__ . '/inc/header.php'; $contacts = [ 'email' => 'Email', 'phone' => 'Telephone' ]; $errors = []; $request_method = strtoupper($_SERVER['REQUEST_METHOD']); if ($request_method === 'Go') { require __DIR__ . '/inc/get.php'; } elseif ($request_method === 'POST') { require __DIR__ . '/inc/post.php'; } require __DIR__ . '/inc/footer.php';
Code language: PHP ( php )
How information technology works.
The $contacts
array is used to generate radio buttons dynamically. In a real awarding, the data may come from a database table or a upshot of an API telephone call.
The $errors
array is used to collect the error letters.
To get the HTTP request method, you admission the 'REQUEST_METHOD'
cardinal of the $_SERVER
array. The strtoupper()
function converts the request method to capital.
$request_method = strtoupper($_SERVER['REQUEST_METHOD']);
Code language: PHP ( php )
The alphabetize.php
file loads the get.php
from the inc
directory if the request is Go. When the course is submitted with the Postal service request, the index.php
loads the postal service.php
from the inc
directory:
if ($request_method === 'GET') { require __DIR__ . '/inc/get.php'; } elseif ($request_method === 'Post') { require __DIR__ . '/inc/post.php'; }
Lawmaking linguistic communication: PHP ( php )
5th, create a form in the go.php
file:
<form activeness="<?php repeat htmlspecialchars($_SERVER['PHP_SELF']) ?>" method="post"> <div>Please choose your preferred method of contact:</div> <?php foreach ($contacts as $key => $value) : ?> <div> <input blazon="radio" proper noun="contact" id="contact_<?php echo $key ?>" value="<?php echo $fundamental ?>" /> <label for="contact_<?php echo $key ?>"><?php echo $value ?></label> </div> <?php endforeach ?> <div> <small course="error"><?php echo $errors['contact'] ?? '' ?></small> </div> <div> <button type="submit">Submit</push> </div> </form>
Code language: PHP ( php )
The following code uses a foreach
argument to generate a radio group from the $contacts
array:
<?php foreach ($contacts as $key => $value) : ?> <div> <input type="radio" name="contact" id="contact_<?php repeat $primal ?>" value="<?php echo $central ?>" /> <label for="contact_<?php echo $primal ?>"> <?php echo $value ?> </characterization> </div> <?php endforeach ?>
Code language: PHP ( php )
The following code shows the fault message from the $errors
array. Note that we use the nix coalescing operator (??) which is bachelor in PHP 7+.
The expression $errors['contact'] ?? ''
returns $errors['contact']
if it exists and is not zip or " otherwise.
<pocket-sized form="mistake"><?php echo $errors['contact'] ?? '' ?></modest>
Code language: PHP ( php )
Finally, add the code that handles the Postal service asking:
<?php // sanitize the contact $contact = filter_input(INPUT_POST, 'contact', FILTER_SANITIZE_STRING); // check the selected value confronting the original values if ($contact && array_key_exists($contact, $contacts)) { $contact = htmlspecialchars($contact); } else { $errors['contact'] = 'Please select at least an pick.'; } if (count($errors)) { crave __DIR__ . '/go.php'; } else { echo <<<html You lot selected to be contacted via <strong> $contact</stiff>. <a href="index.php">Back to the grade</a> html; }
Code language: PHP ( php )
How the postal service.php
works.
The filter_input()
part sanitizes the value of the checked radio push. To brand sure the submitted value is valid, we check it confronting the keys of the $contact
array using the array_key_exists()
function.
If the submitted value matches with one of the array keys, nosotros show a message. Otherwise, we add an error bulletin to the $errors
assortment.
In the terminal if...else
statement, nosotros load the grade (get.php
) that shows an error message if the $errors
array is non empty. Otherwise, nosotros evidence a confirmation bulletin.
Summary
- Use the input with
blazon="radio"
to create a radio push. - Use the same name for multiple radio buttons to define a radio group.
- Get the value of a checked radio via the
$_POST
(or$_GET
) variable, depending on the asking method.
Did y'all find this tutorial useful?
Source: https://www.phptutorial.net/php-tutorial/php-radio-button/
0 Response to "How Many Radio Buttons From the Following Code Can Be Selected at Any Given Time?"
Post a Comment